Unravel the technical language of software development
Do you work with developers, or do you simply want to gain a better understanding of the technical language of software development? This article provides a simple overview of some of the terms frequently used in the industry.

Christopher St-Pierre
September 5, 2023
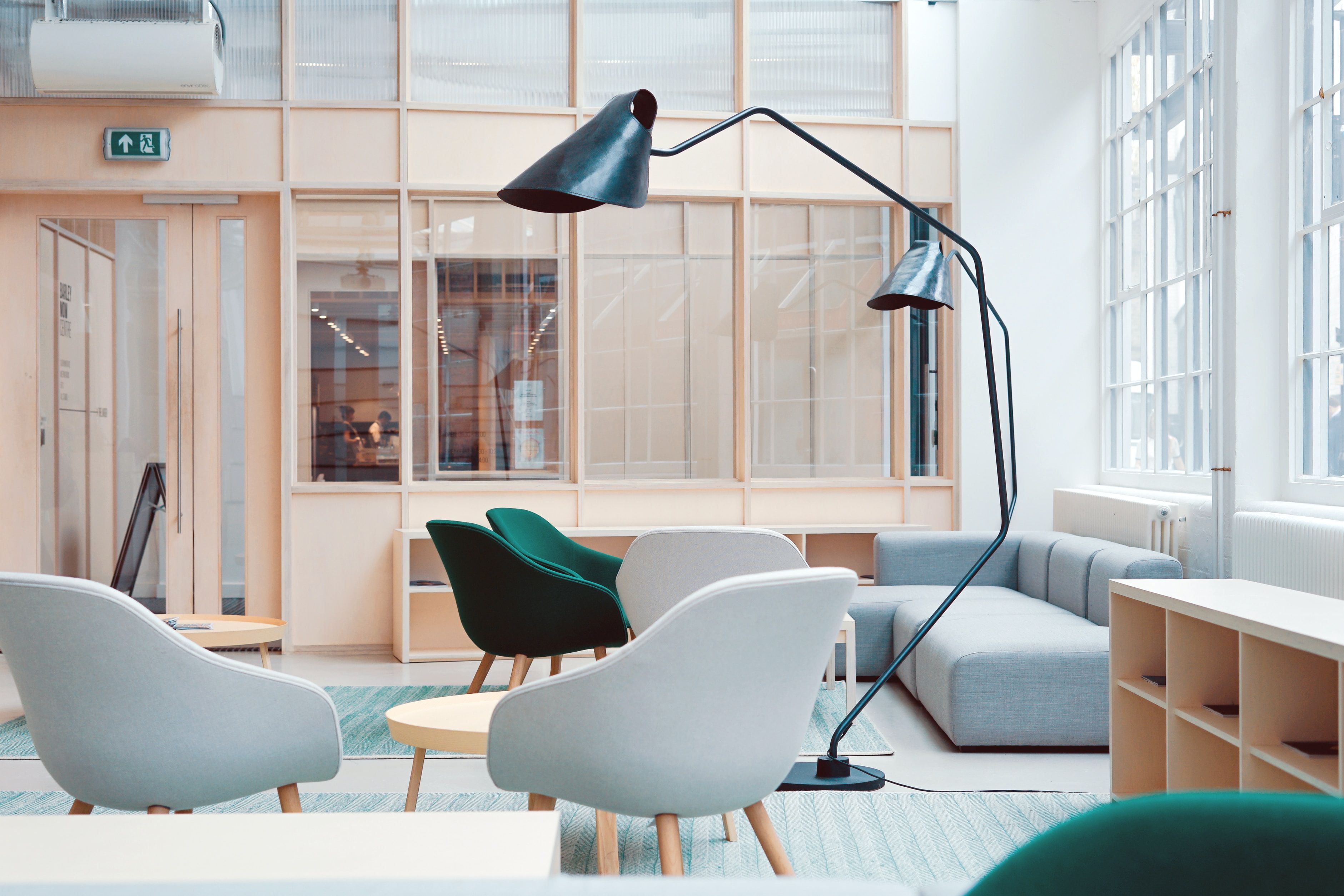
Why should you read this article?
If you work with a team of developers, it's likely that you'll be confronted with technical terms that you may not be familiar with. Rest assured, it's perfectly normal not to know this lingo if you don't work in the industry. Nevertheless, if you're planning to work closely with a software development team, a few basic technical notions could come in very handy!
In this article, we'll give you a simplified introduction to the most important terms in the language of developers. We'll cover concepts such as "frontend" and "backend", "API", and much more, to make sure you're well-equipped for your next technical discussion.
Here's a list of the topics we'll be covering. Feel free to read them all, or to choose the ones that interest you. Happy reading! 😉
Table of contents
- Frontend vs Backend
- UI - User Interface Design
- Code
- Type of solutions
- Data
- Database
- API
- Artificial intelligence
- Hosting
- Cloud
- Project management
- Business Rule
Frontend vs Backend
If you're considering programming services for your project, it's important to understand the terms "frontend" and "backend". These concepts are essential to understand when developing applications and websites. I'm going to explain them in a simple way so that you can have a clear vision of what they mean.
Frontend: What you interact with
Imagine you're looking at a website or an application. All the elements you can see and interact with are part of the user interface and make up the frontend. Buttons, colours, images, links, texts you read - all these are part of the frontend. You could also say it's the design layer of your site or application. It's like the facade of a building, what you notice first and interact with directly. If the frontend itself was a house, it would include the walls, doors and windows that you can see and touch.
Backend: The invisible brain
Now let's move on to the backend. Imagine yourself sending a message on an application like Messenger. You see the messages you and your friend are exchanging. None of this would work without the backend, the brain of the application. The backend manages everything you don't see directly, and contains a set of logical rules that enable actions to be carried out correctly in a system. For example, when you send a message, the backend might take care of retrieving the conversation data, checking whether you're friends with the recipient of the message, ensuring that the message is secure and legitimate, and then forwarding it to your friend. If the frontend was a house, the backend would be the complex set of wires, pipes and systems that keep the house running efficiently.
Frontend and Backend Working Together
Now imagine a team: frontend and backend working together to deliver a seamless user experience. It's quite rare for an application to have a frontend without a backend, or a backend without a frontend. When you send a message, you use the frontend interface to compose it, but it's the backend that contains the logic to deliver the message correctly and securely. They are a dynamic duo working in harmony to deliver a smooth user experience.
Client and server: Interwoven Worlds
When you hear the terms "client" and "server", it's natural to think that "client" refers to you, the user. However, in the language of software development, these terms have a different meaning. The "client" actually represents the frontend, the part you use directly. The "server", on the other hand, corresponds to the backend, managing everything that happens in the background.
In short, the frontend is the visible, interactive part, while the backend manages behind-the-scenes operations to guarantee security and functionality. Together, they create a coherent, fluid user experience. If you have any questions about how these two elements interact in the development of your project, don't hesitate to ask our programming experts.
UI - User Interface Design 🎨
When we first talked about the frontend, we mentioned its link with website design. Indeed, user interface (UI) design is an essential activity when creating the frontend of an application, but this concept is much broader.
Think of it as the thinking behind how the site or app is dressed up and organized so that you can easily interact with its elements. This includes everything from the buttons you click to the images you see, and even the way all the elements move and react when you use it. In a nutshell, user interface design aims to create an intuitive and aesthetically pleasing experience for the user.
Responsive Design
Responsive design is like having an outfit that fits your body perfectly, no matter what your size or shape. When we talk about "responsive" design for a website or application, it means that the user interface is designed so that its appearance and the layout of its graphic elements automatically adapt to different devices, such as phones, tablets and computers.
Think of it this way: picture a website that looks great on your computer. When you view it on your phone, responsive design ensures that elements rearrange themselves to fit the smaller screen. Buttons, images and text are all arranged so that you can see and use them comfortably.
A common example is the menu on a website. Often, the same website menu displayed on a computer screen will look completely different on your phone. On the computer, we generally see all the first-level links horizontally, whereas on mobile we'll see the 3 lines (hamburger menu) that allow us to open the menu vertically. Below, you can see the difference between the computer and mobile versions of our site.

vs
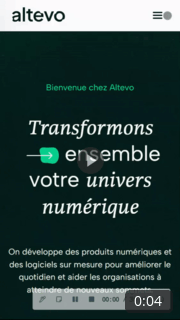
Mobile-First
The concept of "mobile-first" means that when designing a website or application, the user interface is initially designed for mobile users, then adapted for the computer version. This approach is generally used when we know that the website will be consulted mainly by users on their phones.
UX - User Experience Design
Another crucial dimension of frontend design is user experience, also known as UX. This notion refers to the concept of ergonomics, and especially to the way you feel and interact with an application or website when you use it. Imagine walking into a store: the atmosphere, the layout, and the ease of finding what you're looking for all contribute to your experience. UX works the same way for applications and websites.
Think of it as if you were the director of your own online experience. Just as we adjust lights and decor to create a pleasant atmosphere in a store, web designers use UX to make your digital experience enjoyable. This includes small details like changing the appearance of a button when you hover over it, displaying loading icons when there's a wait, or adding animations to show that you've completed an action. Imagine you're on a website and you're looking for the store's phone number, and it's located on a subpage of a subpage. You're going to waste a lot of time finding what you're looking for, and you're not going to enjoy the experience. To counter this, we could put the contact number directly in the menu or at the bottom of all pages.
In other words, UX design is all about identifying and solving users' problems, so that they can use your application or website as pleasantly and efficiently as possible. One way of doing this is to carry out user observation sessions, to understand how people use the application and what difficulties they encounter, with the aim of identifying ways of improving the user interface.
Code 📝
Code is basically what allows developers to build softwares or applications. In order to develop an application, we use programming languages with which we write instructions to determine all the application's business or display logic. In a way, code is how humans communicate with computers in an understandable language.
If we compare it to a cooking recipe, code would be the sequence of instructions which, when executed in the right order, enables us to prepare a delicious meal. Thus, the developer writes lines of code to tell the computer how to build the application. Then, when the application is run on your computer, browser or mobile device, the processor on your device reads these instructions to bring the application to life.
using System; class Programme { static void Main(string[] args) { int age = 20; // Replace this with the user's age if (age >= 18) { Console.WriteLine("You can enter the site."); } else { Console.WriteLine("Sorry, you must be at least 18 years old to enter the site."); } } }
The entire code of an application is the source code. This is what you should be given at the end of a project. You might hear the terms "source code manager", "git" or "repository". All these designate the place where the source code is kept. This allows us to see what changes have been made by whom and when, a bit like a Google Drive Storage, but for code.
Programming Languages
As mentioned earlier, programming languages are the languages with which applications are coded. They enable us to give instructions to a computer. To draw a parallel, we construct a text with words in a language like English. These words, which follow each other in a certain order, will form sentences and a meaning for the reader. The principle is the same for programming languages. They define a sequence of terms (like words) which, when put together, enable the computer to understand what it needs to do. There are many different programming languages, such as JavaScript, C#, Python, HTML and so on. Different languages are used depending on the type of solution we're developing. Some are specific to the frontend, others to the backend, others to databases, etc.
Bug
A bug, in the world of computing and software development, refers to an error, defect or problem in a software program which prevents it from working as expected. Bugs can cause unwanted behaviours, crashes, display errors or other problems that cause the application or system to become unusable or unstable.
Bugs can occur for a variety of reasons, including programming errors, complex interactions between different components of an application, unexpected conditions or unanticipated scenarios. Software developers strive to identify and resolve bugs in order to improve the quality and stability of applications.
To detect and fix bugs, developers carry out in-depth tests, use debugging tools and work cooperatively to identify the source of a problem. Once the bug has been identified, developers fix the affected code to eliminate the error and ensure that the application operates correctly.
Tests
Imagine you're building a house. Before you move in, you want to make sure that everything in the house works properly and is in good condition. That's how testing works in software development.
When we create an application, we want to be sure that it performs as expected. Testing is like checking your house to make sure the lights come on, the taps run and the doors close properly.
Software testing allows us to spot potential errors or problems in the program. For example, we might check that buttons work when clicked, that information is displayed correctly on the screen regardless of the device used, and that the application works swiftly.
Just as you would inspect a house before moving in to make sure that everything is ready, we carry out tests to ensure that the application is ready for you and your users to use. This helps us deliver a high-quality experience, free from errors and annoying problems.
There are different types of testing. Some can be automated, so we can run them before delivering a new version to users. Other tests are harder to automate and require a team to carry out a series of manual tests.
Depending on the type of application and the risks involved, it may be necessary to carry out complete phases of testing to minimize the impact.
Type of Software Solutions 💻
When exploring the field of software solutions, it's common to come across a variety of terms that can be confusing. Understanding the nuances between these terms is essential, as it will enable you to choose the most suitable solution for your needs. Here's a clear overview of the main types of software solutions.
Websites
A website, whatever its nature, is a page that you can access through a web browser such as Google Chrome or Safari. Unlike applications, websites are generally used to present information, products or services. They're like a virtual showcase for a company or project.
Display Site: for Online Visibility
Display sites are ubiquitous on the market. Almost every company has one. They provide online visibility and showcases a company's know-how. The site you're currently visiting is an example of a display site. Its purpose is to present our services, facilitate contact with potential customers and provide useful resources for starting up projects.
CMS vs. Static Site: Flexibility vs. Simplicity
Display sites can be equipped with a CMS (content management system) or be static. CMS allow site owners to easily edit content, add new pages and customize the look and feel without the need for technical expertise. Often, you'll hear WordPress mentioned when people refer to a CMS (that's because it's the most used, but that doesn't mean it's the best). On the other hand, updating static sites often requires you to modify the code directly, which requires technical knowledge. But, in return, it can be built faster and generally costs less.
E-Commerce: Selling Online
E-Commerce, or electronic commerce, refers to sites whose purpose is to sell products or services. A famous example is Amazon. Other forms of E-Commerce sell services (Fiverr), online courses (e.g. Udemy), reservations (e.g. Airbnb), etc.
Applications : Beyond Mobile Apps
Application : An application is not necessarily a mobile app. It can also be a web application. The term application is what brings the two together. In an application, whatever its subtype, there's a certain logic that's tailored to the functionalities it offers. For example, on Messenger, you'll find features that let you send and receive messages or add friends, and in another one, like your banking application, you'll be able to deposit a check, make a transfer, check your account balances, and so on. These are two applications with completely different actions, which defines their uniqueness. In other words, applications are designed to perform specific tasks and includes and unique scenarios of user interactions. On the other hand, on display websites, you'll often see the same information: service page, team presentation, contact page, and so on.
Here are a few variations of the word "application" which you might encounter.
Web application: A web application is one that can be accessed via a web browser. Users don't need to download or install any software on their device, as it works online. Examples include social media sites like Facebook and e-mail services like Gmail. There's also Google Maps, Wikipedia, YouTube, Pinterest, Canva and more.
Mobile application: A mobile application, commonly referred to as an app, is specifically designed for use on mobile devices such as smartphones and tablets. Mobile applications can be downloaded and installed from application stores such as Apple's App Store or the Google Play Store.
Desktop application: A desktop application is a software program designed for use on a desktop or laptop computer. Unlike web applications, desktop applications generally need to be installed on the local device. Examples of desktop applications include Microsoft Word and Adobe Photoshop.
Application SAAS : A SAAS, literally Software As A Service, is a web application with a subscription model where users pay periodically to access the application. SAAS providers manage updates, maintenance and technological infrastructure. Here are a few examples of SAAS:
- Microsoft 365
- Dropbox Business
- Netflix
- Spotify
While all SAAS are web or mobile applications, it should be noted that not all web applications are SAAS. In short, a web application is a broader term that refers to any application accessible via a web browser, while SAAS refers specifically to the software distribution model where an application is offered as an online service with a subscription model.
Data 📄
Data is information, facts or details that can be recorded and stored for later use, analysis or reference. Data can be numbers, text, images, video or any other element that represents part of the information.
Let's take a few concrete examples:
- Your last name, first name and address are data that identifies who you are.
- A friend's phone number is data you can use to contact them.
- The daily temperatures recorded over a week are data you can use to understand weather trends.
- The number of likes on a social networking publication is data that indicates how users interact with the content.
In short, data are the things that contain information and facts that we can use to make decisions, learn new things or simply understand the world around us.
Database 🗄️
A database, as the name suggests, is used to store and organize data. Think of all the information you might have on your customers, your products or your transactions. Instead of keeping it all in scattered files, a database lets you store and organize this information in a structured way. This makes it easier to find, update and manage this data. A simplistic example of a database could be Excel. There could be one table for clients, another for orders, and you could make relationships using Excel. A database is essentially the same thing, but it allows you to manage much more data (millions), much more quickly and with considerably less effort than using Excel.
When we talk about a database, we often refer to tables, which can be summarized as categories of information (customer, order, supplier, etc.), and columns, which are the data contained in these tables (customer number, customer name, e-mail address, address, etc.). A table generally has several columns.
Databases are managed by a database engine. There are several of them (you may have already heard of MySQL, PostgreSQL or Microsoft SQL Server), but we won't go into details in this article.
API ⚙️
API is a widely-used programming term. It stands for Application Programming Interface. In simple terms, an API is a component that handles data exchange and processing between different parts of an application. We defined the difference between frontend and backend above. Generally speaking, an API refers to a type of backend. The API may, for example, be responsible for storing the information it receives from the frontend in a database, performing processing, sending e-mails or notifications, and so on. In fact, the API is generally where the business logic is found.
When developing a web application, we generally develop the API that goes with it. We create functionalities on this API that can be used by the frontend (whether it's a mobile application or a web application), as we explained in the backend section. The advantage of web APIs is that they allow us to have a unique backend, detached from the frontend, which can be reused if we want to develop a mobile application or another application linked to the same system. This is what happens with Facebook, for instance. They provide a web application and a mobile application, both of which interact with the Facebook API. So no matter which platform you're connected to when you read a Facebook post, the data processing logic remains the same and is located in one and the same place (see image below).
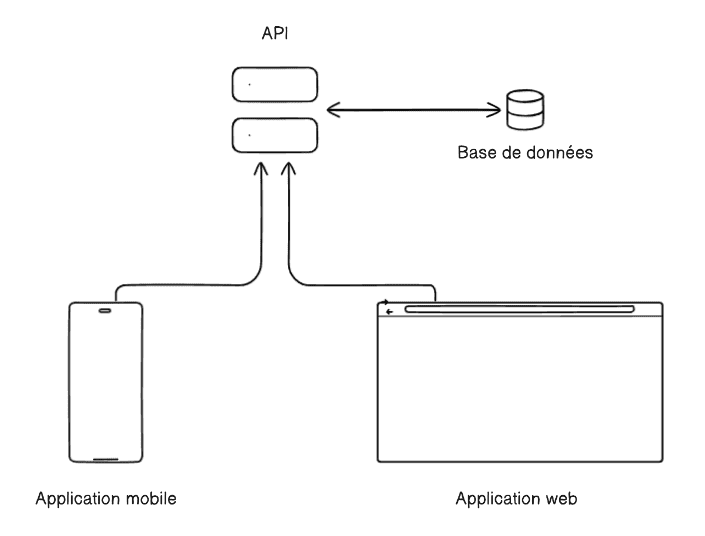
Private API vs. Public API
A private API is actually an API developed specifically for your applications and not intended or designed to be used by other systems.
A public API, on the other hand, is designed to be accessed by other systems. For example, one of the most widely used APIs is certainly provided by Google Map, which can be used, among other things, to provide the distance between two locations, driving directions and so on. Therefore, if in your application you want to display the number of kilometres between a client's place of business and your warehouse, you can use this API so you don't have to develop all this logic on your own. Another example might be the Google review API. Let's say you want to show the reviews that customers have written for you in Google directly on your website, you could use the Google Review API to retrieve those reviews and display them on your site. In short, a public API enables you to connect several existing systems or services together so that you can take advantage of all their functionalities.
Artificial Intelligence 🤖
Very much in the spotlight this year, this topic could require an article of its own. Artificial intelligence (AI) is a field of computer science that aims to create machines capable of performing tasks that would normally require human intelligence. Rather than simply executing programmed instructions (as in a traditional system), AI systems are designed to mimic some of the human cognitive abilities, such as language comprehension, problem-solving, decision-making and even learning from experience.
AI uses a variety of techniques and methods, some of which are inspired by the way the human brain works. Here are some important areas and concepts of artificial intelligence:
-
Machine Learning (ML): An approach to AI where systems learn from data rather than being explicitly programmed. Using complex statistical calculations, they identify patterns and trends in the data to improve their performance over time. There are several algorithms such as Neural Networks, that are used to solve complex problems such as image recognition, automatic translation and many others.
-
Natural Language Processing (NLP) : A branch of AI that focuses on understanding and generating human language by machines. Chatbots and voice assistants are examples of NLP applications.
-
Computer Vision : AI can be used to analyze and understand images and videos, enabling applications such as facial recognition, object detection and autonomous driving. The aim of artificial intelligence is to enable machines to perform tasks that could not be easily achieved by standard programming, which can include solving complex problems, making decisions, analyzing large amounts of data and much more.
For example, AI could be used in your applications to understand texts that customers send you, detect document types when submitting a form, detect the mood of your customers when they send you a message, suggest content when you write a text, and so on. For our part, we recently used a NER (named entity recognition) service to detect key information contained in a text for one of our projects.
Hosting 🏢
When you launch a website or an online application, you need to store it somewhere so that others can access it via the Internet. Imagine your website is like a house, and you want to show it off to everyone. You need a place to put that house and make it accessible to everyone.
Hosting is like renting land to build your house. You pay a hosting company to put your website or application on its servers, which are special computers designed to store and distribute web sites or applications.
When someone wants to visit your site, they type the address into their browser (the domain) then the browser connects to the hosting servers where your site is stored. The servers in turn send the information needed to display your site on the user's screen.
In other words, hosting is like renting a space on the Internet to store your databases and web applications, making it accessible to everyone. This ensures that your site is online and visible to your users.
Finally, it's worth mentioning that hosting can be done on the cloud or directly in your company's server room. We'll talk more about this in the next section.
Deployment
Deployment is simply the act of making an application or website available on the environment where it is hosted. This generally involves compiling and copying new versions of the code to your hosting provider.
Environments
There are several types of hosting environment.
Production : Contrary to what the word production might suggest, it doesn't refer to something in development. The production environment is where the application is accessed and used by end-users. It's the final stage in a deployment cycle. When deploying to this environment, we need to ensure that the site has been tested and is ready for use.
Staging : The staging environment, also known as pre-production, is an environment that closely resembles the production environment, but is used to carry out final tests before deploying the application on the real production environment. This is a useful step to check that everything is working properly before bringing the application online.
QA (Quality Assurance) : The Quality Assurance (QA) environment is used to further test the application to ensure that it runs correctly and is free from bugs and errors. QA teams carry out in-depth tests in this environment to guarantee the quality and stability of the application. So, when someone finds a bug, they tell the developers what the problem is so that it can be fixed, and then a new version is deployed on the QA environment to confirm that the problem has been corrected.
Development : The development environment is where developers initially create and test the application's source code. This is often the developer's computer.
Domain Name
A domain name is like your website address on the Internet. It's what people type into their browsers to find you online. A bit like your home address, which lets people know where you live without having to enter GPS coordinates (longitude and latitude), a domain prevents people from having to type in the IP address of servers by hand to access a website or application. For instance, to access our website, all you have to do is type altevo.ca instead of 76.76.21.21 in your search bar. This makes the domain much easier to remember and more aesthetically pleasing than the IP address.
Cloud ☁️
The "cloud" concept can be compared to an online service where you can get everything you need without owning the equipment yourself. Imagine being able to rent special tools whenever you need them, instead of buying them and storing them at home. Similarly, in the IT world, the cloud lets you access computers, programs and data via the Internet, without having to own the equipment physically.
Think of the cloud as a virtual place where everything is stored and managed online, on remote computers. Instead of keeping servers and software in your home or business, you can "rent" them remotely.
Cloud Computing
You may need to work with Cloud Computing services to develop your applications. There are 3 major providers in this field: Azure (Microsoft), AWS (Amazon), GCP (Google). These services enable you to do a whole lot of things, including hosting your applications, processing data, managing user authentication, sending notifications, managing databases and so on. Just about anything that can be installed on a server is available in the cloud. The providers all work in much the same way: you pay by subscription to services or by usage. So, if you deploy your API on this service, you benefit from many advantages such as security, redundancy, scalability (the ability of an application to increase its resources according to demand, for example to respond to a large volume of users). What's more, you don't have to manage this infrastructure and install services on servers yourself.
In short, a Cloud Computing service means you don't have to host your websites/infrastructure (APIs, databases, etc.) in your company's own server room. You delegate hosting to these services in exchange for a monthly payment.
Cloud Storage
Many people also refer to the Cloud when they talk about file storage locations. In fact, several services enable you to manage files on servers accessible from anywhere in the world. This means you can log in from any device and view your files wherever you are. Here are just a few examples: OneDrive, Google Drive, iCloud (Apple) and Dropbox.
There are also cloud storage solutions for businesses, such as Google Workspace and SharePoint.
Project Management 📽️
Scrum (Agile)
Scrum is a project management methodology often used in software projects. The method is quite comprehensive, and we could talk about many aspects in a full article. But, to briefly summarize, the idea is to plan development phases called sprints, where developers prioritize tasks with the customer and ensure that by the end of each iteration (sprint) the result is a usable product for the customer. There are many advantages to using this method, since it puts the customer at the center of the project's development, and his feedback is important at every stage. They will be involved in planning, reviews and demonstrations. For this method to work, the customer must have a considerable amount of time to devote to the project.
In addition to Scrum, there are many other methods in the Agile family, such as Kanban or hybrid methods. Each development organization generally decides how it wishes to proceed.
Jira
Jira is a software project management system. It is used to record all project requirements and divide them into small tasks (also called User Story) that can be prioritized and organized over time. Chances are your software development team uses this tool or an equivalent. It organizes the project and facilitates communication. This software is directly compatible with scrum / kanban methods and helps organize the process (plus it's a SAAS 😉).
User Story
Earlier, we explained that an agile project is divided into several small tasks. These tasks are often formulated in the form of user stories. A user story is a user-friendly way of describing what a user wants to achieve by using an application or website. Think of each user story as a short narrative about what the user wants to do and why. This helps the development team understand exactly what you want the application to do, and what features need to be integrated into it. Here's an example: As a prospective buyer, I want to be able to consult customer reviews of the products, so I can make an accurate purchasing decision.
Acceptance Criteria
Acceptance criteria refer to checklists to ensure that the work done on a particular feature or task meets your expectations. These are the points that must be integrated into the software and validated during QA before a feature can be considered delivered. In the example above, here's what the acceptance criteria could have been for the user story:
- On each product page, customer reviews will be displayed with an overall star rating and a comment.
- Reviews can be sorted by relevance, ascending and descending rating.
- Each review will display the date it was published, the author's name and its rating.
- Reviews are submitted by authenticated clients who have actually purchased the product.
- Reviews containing inappropriate language can be flagged by users and will be subject to moderation.
Business Rule 💼
A business rule is an instruction or condition that guides the way a company operates or makes decisions. It's a bit like the rules that help an organization maintain specific standards or procedures. For example, a business rule might be "All users must be at least 18 years old to create an account."
It's important to define these rules carefully, as they influence the application's behaviour and will need to be considered during development.
Take Action with Altevo!
We hope this guide has strengthened your understanding of the fundamental technical terms widely used in the world of software development. We recognize that these concepts may seem daunting to those unfamiliar with the domain, but thanks to the knowledge you've acquired, we're confident you'll tackle the rest with ease.
At Altevo, we believe in a human approach and are committed to making software development approachable to everyone, guiding and involving you every step of the way. Our dedicated team is ready to support you in your projects, whatever your level of technical knowledge. Whether it's answering your questions, turning your software product ideas into reality, or overcoming challenges specific to your organization, we're here to help.
To find out more about our development services and how we can help you realize your projects, please don't hesitate to contact us using this form. Moreover, if you already have a vision in mind and would like us to work together to make it a reality, book a free discovery meeting today.
Together, let's elevate your business ! 🚀
Article photo by Toa Heftiba on Unsplash
About us
Altevo is a team of passionate developers combining their talents to build tailor-made web applications and software solutions. Guided by a strong human culture, our software engineering expertise allows us to help our clients elevate their business.
Have you got a project in mind, or just curious to find out more? Let's talk!